Productivity tools
Objectives
Know about tools that can help you spot code problems and help you following a consistent code style without you having to do it manually.
Get an overview of AI-based tools and how they can help you writing code.
Instructor note
Demo/discussion: 20 min
Linters and formatters
Linter: Tool that analyzes source code to detect potential errors, unused imports, unused variables, code style violations, and to improve readability.
Formatter: Tool that automatically formats your code to a consistent style, for instance following PEP 8.
In this course we will focus on Ruff since it can do both checking and formatting and you don’t have to switch between multiple tools.
Linters and formatters can be configured to your liking
These tools typically have good defaults. But if you don’t like the defaults, you can configure what they should ignore or how they should format or not format.
Examples
This code example (which we possibly recognize from the previous section about Profiling and Tracing) has few problems (highlighted):
import re
import requests
def count_unique_words(file_path: str) -> int:
unique_words = set()
forgotten_variable = 13
with open(file_path, "r", encoding="utf-8") as file:
for line in file:
words = re.findall(r"\b\w+\b", line.lower()))
for word in words:
unique_words.add(word)
return len(unique_words)
Please try whether you can locate these problems using Ruff:
$ ruff check
Next, let us try to auto-format a code example which is badly formatted and also difficult to read:
import re
def count_unique_words (file_path : str)->int:
unique_words=set()
with open(file_path,"r",encoding="utf-8") as file:
for line in file:
words=re.findall(r"\b\w+\b",line.lower())
for word in words:
unique_words.add(word)
return len( unique_words )
import re
def count_unique_words(file_path: str) -> int:
unique_words = set()
with open(file_path, "r", encoding="utf-8") as file:
for line in file:
words = re.findall(r"\b\w+\b", line.lower())
for word in words:
unique_words.add(word)
return len(unique_words)
This was done using:
$ ruff format
Type checking
A (static) type checker is a tool that checks whether the types of variables in your code match the types that you have specified.
Integration with editors
Many/most of the above tools can be integrated with your editor. For instance, you can configure your editor to automatically format your code when you save the file. However, this only makes sense when all team members agree to follow the same style, otherwise saving and possibly committing changes to version control will show up changes to code written by others which you possibly didn’t intend to make.
Integration with Jupyter notebooks
It is possible to automatically format your code in Jupyter notebooks! For this to work you need the following three dependencies installed:
jupyterlab-code-formatter
black
isort
More information and a screen-cast of how this works can be found at https://jupyterlab-code-formatter.readthedocs.io/.
Integration with version control
If you use version control and like to have your code checked or formatted before you commit the change, you can use tools like pre-commit.
AI-assisted coding
We can use AI as an assistant/apprentice:
Code completion
Write a test based on an implementation
Write an implementation based on a test
Or we can use AI as a mentor:
Explain a concept
Improve code
Show a different (possibly better) way of implementing the same thing
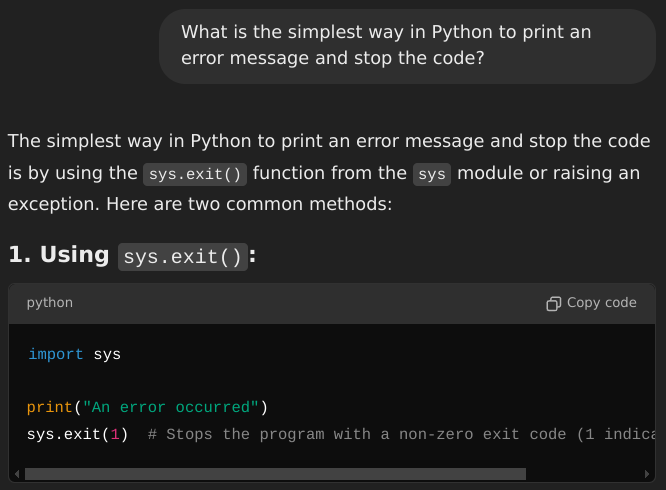
Example for using a chat-based AI tool.
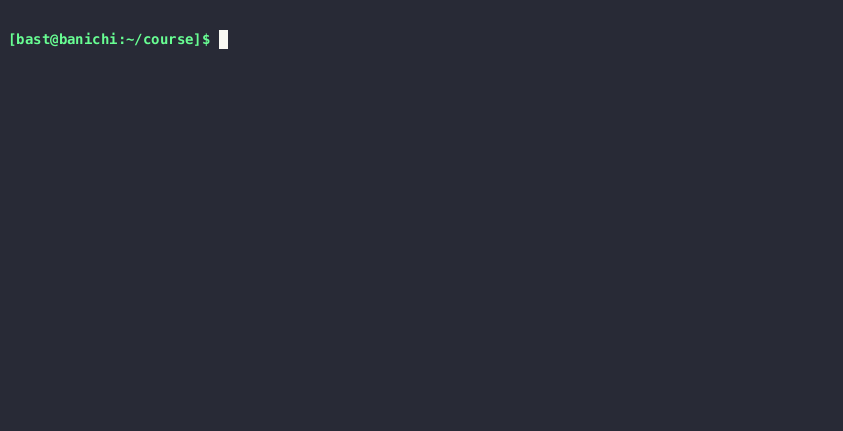
Example for using AI to complete code in an editor.
AI tools open up a box of questions
Legal
Ethical
Privacy
Lock-in/ monopolies
Lack of diversity
Will we still need to learn programming?
How will it affect learning and teaching programming?